Restlet 2.0 sample application with annotations
Friday, October 23, 2009
The new version of Restlet, 2.0, uses Java annotations to make web service development easier than it was with version 1.1. However, these annotations are not based on the JAX-RS specification. They are similar, but they are not the same.
In
Chapter 6, I cover in detail the web component portion of the Restlet framework, versions 1.1 and 2.0. For this entry, however, I will just give an example of a full RESTful web service implementation using Restlet 2.0 that runs as a standalone Java application (I cover this topic in
Chapter 9).
The Restlet framework, aside from a Java web container component, offers a library for standalone web application development. With it, you can create desktop applications that don't require a full Java web container such as Tomcat or JBoss (though, technically, the standalone library provides a deployment-free HTTP server).
A full listing of a Restlet web service looks as follows:
import org.restlet.Server;
import org.restlet.data.Protocol;
import org.restlet.resource.Delete;
import org.restlet.resource.Get;
import org.restlet.resource.Post;
import org.restlet.resource.Put;
import org.restlet.resource.ServerResource;
public class StandAloneServer extends ServerResource {
public static void main(String[] args) throws Exception {
new Server(Protocol.HTTP, 8006,
StandAloneServer.class).start();
}
@Get
public String handleGet() {
return "HTTP GET.";
}
@Post
public String handlePost() {
return "HTTP POST.";
}
@Put
public String handlePut() {
return "HTTP PUT.";
}
@Delete
public String handleDelete() {
return "HTTP DELETE.";
}
}
The
main()
method instantiates an
org.restlet.Server
object that listens to HTTP requests on port
8006
. This takes care of the HTTP request listening.
What about the specific HTTP request methods?
This is where the Restlet annotation functionality comes into play. Servicing specific HTTP requests takes nothing more than coding and annotating a method for each HTTP request, as follows:
@Get
- for resource retrieval.@Post
- for resource creation.@Put
- for resource updates.@Delete
- for resource deletion.
The name of each annotated method is arbitrary, and this is represented in my example above. For instance,
handleGet()
handles GET requests,
handlePost()
handles POST requests,
handlePut()
handles PUT requests, and
handleDelete()
handles DELETE requests.
Each of these request handlers returns a
String
representation as the response. For a real example, however, you should be returning XML or JSON structures that are specific to your problem domain.
Assuming that your Java source file is called
StandAloneServer.java
, you can compile this application with the following command:
javac -classpath RESTLET_HOME/lib/org.restlet.jar StandAloneServer.java
To run the application, you would use the command:
java -classpath RESTLET_HOME/lib/org.restlet.jar StandAloneServer.java
To access the web service, just point your testing client to the URI
http://localhost:8006/
. For a GET request, you can use your web browser. For example, using FireFox, a GET request's response looks as follows:
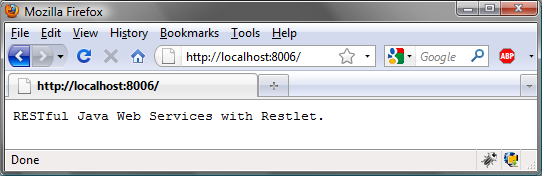
To test the requests POST, PUT, and DELETE, you will need a more sophisticated web client, as web browsers can't generate PUT or DELETE requests. If you don't have a client that can generate these requests, you can use my Java Swing client (full code listing is in
Chapter 2). A PUT request using this client looks as follows:
Comments: