Google App Engine and Python Learning from a Java Developer's Point of View
Monday, February 23, 2009
I've had a Google App Engine account for a while now, but I had never created anything of substance until this weekend. I have always liked Google's concept of forgetting the hard and expensive parts of deploying applications on the web and concentrate on the actual business rules. Not knowing if the promise would apply to me, I started to seriously look at this architecture a couple of days ago.
One of the reasons I hadn't coded an application in this architecture is because I didn't know Python--Python being the only supported programming language so far. I'm no stranger to learning new languages and I actually like trying new things, so being sick (a head cold) and unable to sleep, I actually decided to learn Python to create a real application. This was not such a good idea, as I didn't sleep at all last night, because I was really exited about my new toy.
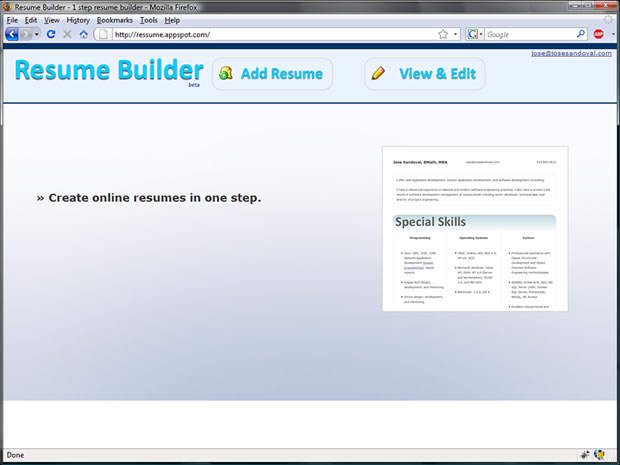
I'm writing this entry in case you are in the same position I was: you are a developer of large scale system and would like to try something new--a way to recharge the batteries, if you will. In this post, I can only answer 2 questions: do I like python? And what is it like for a developer with some experience with other OO languages to come fresh into this scripting world?
First, I like Python. I mean, I don't love it but I don't dislike it, as it's just another implementation technology--I don't really care what I code in: picking up the syntax of a new language is not that hard; finding interesting problems to solve is the hard part.
Having being in the trenches for 2 days straight, I think it's a nice OO language, but I'm used to "real" programming languages with semicolons, curly braces, and strongly typed variables. If you are old school, Python can seem, at first, a bit toysy and unsophisticated (it isn't, of course).
My experience with the language was minimal, and I had only skimmed through a couple of books at the local bookshop. I had also read Google's intro for working with their architecture. It took me a couple of hours to start actually programming, not just copying and pasting code from one one window to the next. At the end of the first day (6 hours or so), I got the hang of it, and the language has grown on me.
I have to admit, however, that I like it because of the applicability to the Google App Engine environment. I don't know of many large Python projects, but maintenance of a large scale system is probably hard. It's true that you can layer your development logic, but newer web applications don't require 50+ engineers to complete, and things can get messy fast. Mind you that we can write messy code in any language. Nevertheless, I like to try to fake a semblance of control. Well structured code is something I take to heart, as it is a pleasure to read and maintain (even those spaces and tab sizes matter).
Which brings me to Python's coding style. In the Python world
variableNames are not what I'm used to. Method naming conventions follow a very SQL like naming structure with
underscores_every_where. I could just use the style I'm used to, but I can't--like I said, I like to follow proper coding styles for the language I'm using. Because of this, before I did any coding, I read Python's
coding style guidelines.
Not everything about the language is to my liking. One of my biggest pet peeves is the interpreter's strictness of indentation to determine scope of variables and statements. For example, if one line of code is not indented properly, the program where this line comes from will never run. It seems like a silly thing to penalize developers for a missing space. I guess it's one of the reason I like the C/C++/Java/C# type of languages: the braces and semicolons take care of scoping.
So that you don't think I'm complaining because I'm being a big cry-baby, this is an actual class in my application:
class Job(webapp.RequestHandler):
def get(self):
resume_key = self.request.get('resume_key')
resume = db.get(resume_key)
template_values = {'loggedIn': False,
'displayResume': True,
'resume': resume}
path = os.path.join(os.path.dirname(__file__), 'resume.html')
self.response.out.write(template.render(path, template_values))
You would likely spend a lot of time to find the bug in this code, so I'll just tell you. Note the line where
template_values
is defined: I have added an extra space to make my point. This code will not run. Fortunately, you can pick up those bugs if you use an IDE like Eclipse and a development plug-in like
Pydev. (To set up your GAE environment to run within Eclipse, follow these instructions:
Google App Engine & eclipse (PyDev).)
As usual, the going started slow, but after two days I have seen the quantity of usable and working lines of codes increase considerably, and continues to increase. Specially, when I can now shorten lines of code. For example, the above code can be written in 1 line--but I wouldn't recommend it:
class Job(webapp.RequestHandler):
def get(self):
self.response.out.write(template.render(
os.path.join(os.path.dirname(__file__),
'resume.html'),
{'loggedIn': False,
'displayResume': True,
'resume': db.get(self.request.get('resume_key'))}))
Which version did I use in my program? The first one. I'm just making a point that you can do crazy things once you become familiar with the syntax.
Did I buy any Python books for my programming adventure? I didn't. I looked for books; however, I didn't like any of the currently published works: I found them boring. I would have not pick up the language, as quickly as I did, by running silly string splitting examples all day, or counting the keys in a dictionary ADT until sunrise.
If you want to learn Python, you should consider the Google App Engine architecture to play with. It's a complete development platform and you get immediate rewards: you store things into a non-relational database by calling a
put()
method from an inherited Model class; you parse HTTP requests by getting the name/value pairs from the input stream; and you send emails by calling the supplied mail library. I did all of these in my
Resume Builder application, without knowing too much about Python.
As an aside, I've come to know that learning programming can be tedious, and borderlines on obsessive compulsiveness. It's hard to explain, but if you haven't sat in front of a computer for 12+ hours straight writing code, you will not understand the thrill of seeing an application run in front of you. I only mention this because I have never been able to learn coding by reading a book--and why I think the Python books I considered were boring. Mind you that this is my experience, but you could learn anything with very little supervision and just by reading. I'm just saying that I got more out of programming this application and learned a great deal because of the Google App Engine architecture. I don't think I could have got the same gentle introduction of the language from any of the typical Python books. In other words, I learned Python and I am now a believer that Google's architecture is a viable computing platform for real web application development.

About the
Resume Builder: I wanted to play with the latest UI recommendations that people are talking and writing about. I recommend reading the book on the left, if you have any interest in user interfaces for the web. The book is good, but there are no implementation examples in it--to be fair, the book is not an example driven read and the authors make that clear in the introduction. For code samples, you will have to look around and code things yourself. Moreover, you will have to use
scriptaculous or jQuery to implement any of the recommendations; however, you will find that most of the hard work is already done for you with these open sourced libraries.
What does my app do? It's all about inline editing, discoverable elements, and Ajax calls galore. Oh, I also added Google's AdSense to see if it generates any money. Finally, this app does one thing only: you can create your resume in one step, and you can then send a link of it for people to see it. For example, this is my
resume.
Comments:
For your mis-indentation example, the Python interpreter should report the problem. I put your code in an x.py file, did an "import x" and got this:
Traceback (most recent call last):
File "x.py", line 6
template_values = {'loggedIn': False,
^
IndentationError: unexpected indent
If AppEngine doesn't report exceptions like this, that's a tool issue, not a language issue.
I'm not disagreeing with you here, and the problem is reported via the IDE. My issue is that from first sight, nothing is wrong with that line.
I do think the language is useful and I'm glad I went through the learning experience.
FYI Google's tool, does report the problem.
Hi Jose. Thanks for an informative article. I found many of your comments and suggestions chimed with my own experience. I am currently trying to find time to get to grips with Google App Engine and learning Python at the same time. I do know Java, and this is now supported in the App Engine, but Python was Google's first choice and it's widely used, so I figure it's worth knowing.
Like you, I prefer to learn by doing, rather than ploughing through a book. That said, I have recently bought the Nov 2009 published O'Reilly book, "Programming Google App Engine", and find this an interesting read, at least the first few chapters (as far as I have got). The book is certainly a big improvement on the other O'Reilly title "Using...." which I found way too slow.
As your post was nearly a year ago, I wonder how you've progressed since then. How's the job market for App Engine skills in the States? I'm in the UK where things tend to lag.